React Native Notes
Table Of Contents
Getting Started
What is React Native?
Setting up the development environment
Expo vs React Native CLI
Core Concepts
Components (Functional & Class)
Props and State
Lifecycle Methods (useEffect and beyond)
UI Basics
Views and Layouts
Styling with StyleSheet
Flexbox in React Native
Navigation
Expo Router & File Based Routing
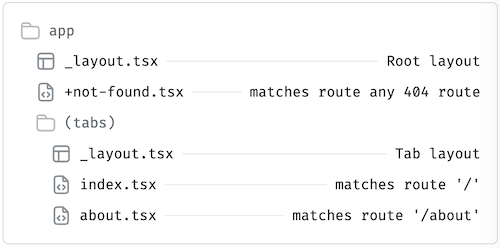
+
Routes: Pages/Screens the user can navigate to.
_
Layouts: Non-navigable components that organize or group routes.
For Example:
app/
_layout.tsx # Shared layout for all pages in this folder.
+index.tsx # Route for the "home or index" screen.
+profile.tsx # Route for the "profile" screen.
+not-found.tsx # Special route for unmatched paths (404 page).
When the app is running:
- Navigating to
/index
will render +index.tsx
wrapped in _layout.tsx
.
- If the user navigates to a nonexistent path,
+not-found.tsx
will be shown.
Stack, Tab, and Drawer Navigators
<Stack>
is a component that helps you manage navigation between different screens in your app. It organizes your app’s screens to function like cards in a deck.
- Each
<Stack.Screen>
in your app is a card/page or group.
- You can “push” a new screen onto the stack (add it to the end of the array, i.e. go to a new page).
- You can “pop” a screen off the stack (remove it from the end of the array, i.e. go back to the previous page).
Tabs are controlled by the group (tab)
. You can create any group using ()
enclosing your group’s directory name. Any new (group)
that you create will need its own _layout.tsx
file inside
Working with APIs
Fetching Data (REST API with Fetch or Axios)
Using AsyncStorage for local storage
Managing State (Context API or Redux)
iOS vs Android Differences
Handling Permissions (e.g., Camera, Location)
Images and Videos
Icons and Fonts
Linking assets in React Native
TextInput Basics
Handling Keyboard Avoidance
Advanced Features
Animations (Reanimated or Animated API)
Gesture Handling (React Native Gesture Handler)
Native Modules and Linking
Avoiding Re-renders
Using FlatList and SectionList Efficiently
Debugging and Testing
Common Errors and Fixes
Testing with Jest or Detox
Deployment
Building for Android and iOS
Using Expo for OTA updates
Publishing to App Stores
Community and Resources
Official Documentation and Guides
Popular Libraries to Know
Staying Up-to-date with React Native